文章目录(Table of Contents)
内容简介
这一篇内容主要介绍C中的指针,主要内容如下:
- 指针的概念及定义:说明,指针变量的类型是指指针所指变量的类型,如 char *point是指point指向的是一个char类型的变量;
- 指针变量作为函数参数:举例,交换两个变量的值;
- 通过指针操作数组;
- 通过指针引用字符串;
举例说明
下面四段代码分别演示上面的四个例子;
指针的概念
- #include<stdio.h>
- #include<stdlib.h>
- /*
- 指针的概念 :
- - 指针里存放的是另一个变量的地址; int *p; p=&a;
- - 指针变量的类型是指 指针所指变量的类型(知道起始地址还要知道总长度)
- */
- int main(){
- int a=1,b=2,c;
- int *point_1,*point_2; //定义指向整数类型的指针变量,这里的*只是表示该变量类型是指针
- printf("a:%d,b:%d\n",a,b);
- point_1=&a; //指针point_1指向变量a的地址
- point_2=&b;
- printf("变量a与b的地址为:%p,%p\n",point_1,point_2); // %p为point的缩写,打印十六进制整数
- c = *point_1;
- a = *point_2;
- b = c;
- printf("a:%d,b:%d\n",*point_1,*point_2); // *point_1表示指针所指地址的值
- return 0;
- }

指针变量作为函数参数
- #include<stdio.h>
- #include<stdlib.h>
- /*
- 指针变量作为函数参数
- - 举例:交换两个变量的值
- */
- void swap(int *a,int *b); // 函数的声明
- void swap(int *a,int *b){
- int temp;
- temp = *a;
- *a = *b;
- *b = temp;
- }
- int main(){
- int c=1,d=2;
- printf("Before exchange : c=%d,d=%d\n",c,d) ;
- swap(&c,&d);
- printf("After exchange : c=%d,d=%d\n",c,d) ;
- return 0;
- }

针对数组进行操作
注意,下面代码中有p=p+1,这里的p=p+1不是单纯的+1,而是因为指针指向int类型,有4字节, 故p+1是在内存中增加4个字节 。
- #include<stdio.h>
- #include<stdlib.h>
- /*
- 通过指针对数组进行操作
- */
- int main(){
- int a[7]={1,2,3,4,5,6,7};
- int *p;
- p = a; // 与 p=&a[0]; 等价; 数组名代表数组首元素地址
- for(p=a;p<(a+7);p++){
- // 这里的p=p+1不是单纯的+1,而是因为指针指向int类型,有4字节, 故p+1是在内存中增加4个字节
- printf("%d\n",*p);
- }
- return 0;
- }
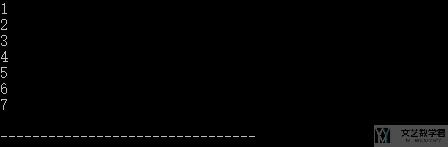
指针引用字符串
下面看一下使用指针来实现自字符串的复制。
- #include<stdio.h>
- #include<stdlib.h>
- /*
- 本篇知识点
- - 通过指针引用字符串
- - 通过指针实现字符串复制
- */
- void string_copy(char *string_1,char *string_2); // 函数的声明
- void string_copy(char *string_1,char *string_2){
- for(;*string_1!='\0';string_1++,string_2++){
- printf("%c ",*string_1);
- *string_2=*string_1;
- }
- *string_2='\0'; // 要在末尾加上结束的标志
- }
- int main(){
- char *p="Hello World!";
- char *q=malloc(15); //分配空间
- printf("%s\n",p);
- // 在输出项中给出字符指针变量名 string, 则系统会输出 string 所指向的字符串第一个字符, 然后自动使 string 加 1, 使之指向下一个字符,再输出该字符;
- // 如此直到遇到字符串结束标志 '\0', 因此再输出是能确定输出的字符到何时结束;
- // 错误写法 printf("%s\n",*p);
- // 使用指针实现字符串的复制
- string_copy(p,q);
- printf("\n%s\n",q);
- free(q);
- return 0;
- }

- 微信公众号
- 关注微信公众号
-
- QQ群
- 我们的QQ群号
-
2021年2月12日 下午4:38 1F
我也写了一篇
但是没这么详细https://www.fivk.cn/archives/123.html
2021年2月15日 下午4:02 B1
@ 梦一帆 写的非常好呀,一起相互学习。